# 2022.09.02
//art shift a 커서 키워서 한번에 지우기
# 다마고치 문제 복습
package day08;
import java.util.Scanner;
class Creature {
// 캐릭터 이름
String name;
// hp
int hp;
// 밥먹은 수
int eatCnt;
// 턴
int turn;
// 변
boolean poo;
public Creature(String name, int hp) {
this.name = name;
this.hp = hp;
}
// 먹기 : 턴을 1중가, hp 2증가
void eat() {
this.turn++;
this.hp += 2;
this.eatCnt++;
}
// 자기 : 3초 동안 잠에 든다. hp 2감소, 턴 2증가
void sleep() {
for (int i = 0; i < 3; i++) {
System.out.println("Zzzz...");
try {
Thread.sleep(1000);// 1000에 1초, 매개변수로 밀리초를 넘겨준다.
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println("잠에서 깼습니다.");
this.hp -= 2;
this.turn += 2;
}
// 대변보기 : 밥을 3번 먹으면 실행, 변을 안치우면 1턴당 hp -1
void poop() {
this.poo = true;
this.eatCnt = 0;
}
// 청소하기 : 대변을 치운다.
void cleanUp() {
this.poo = false;
}
// 상태보기
void printState() {
System.out.println(this.turn + "일차");
System.out.println("이름 : "+ this.name);
System.out.println("Hp : "+this.hp);
}
}
public class Game {
public static void main(String[] args) {
String title = "☆★☆★☆★☆★☆★☆★☆★\n"
+"☆★☆★다마고치☆★☆★"
+"☆★☆★☆★☆★☆★☆★\n";
String mainMsg = "1.캐릭터 선택\n2.종료\n입력 >>";
String charMsg = "1.피카추\n"
+"2.두더지\n"
+"3.긴팔원숭이\n"
+"4.돌아가기\n"
+"입벽\n";
String gameMsg = "1.먹기\n2.자기\n3.청소하기\n 4.상태확인\n입력>>";
Scanner sc = new Scanner(System.in);
int choice =0;
int gameChoice =0;
while(true) {
choice = sc.nextInt();
if(choice ==2) {break;}
choice = sc.nextInt();
if(choice ==4) {continue;}
Creature [] creature = {
new Creature("피카츄",3),
new Creature("두더지",2),
new Creature("긴팔원숭이",4)
};
while(true) {
gameChoice = sc.nextInt();
Creature myChar = creature[choice -1];
switch(gameChoice) {
case 1 :
myChar.eat();
break;
case 2 :
myChar.sleep();
break;
case 3 :
myChar.cleanUp();
break;
case 4 :
myChar.printState();
break;
default :
System.out.println("잘못입력했습니다.");
}
if(myChar.eatCnt >=3) {myChar.poop();}
if(myChar.poo) {
System.out.println("변을 치워야 합니다.");
myChar.hp--;
}
if(myChar.turn >= 10){
System.out.println("*************************");
myChar.printState();
System.out.println("*************************");
System.out.println("*************************");
System.out.println(myChar.hp >=5?"해피엔딩":"베드앤딩");
}
}
}
}
}
# this()
- 자기자신의 생성자를 의미한다.
- 생성자 내부에서만 사용이 가능하며 최상단에 작성해야한다.
//alt 방향키 정이나 앞에 있던 곳으로 이동한다.
받는 쪽 : 메게변수 / parameter
보내는 쪽 : 인수, 인자, argument
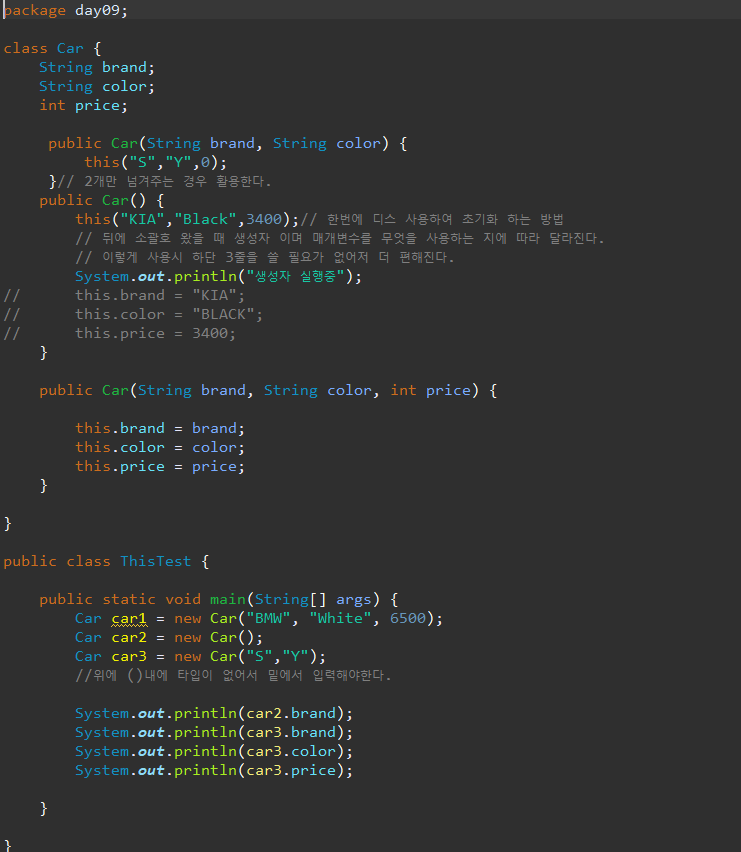
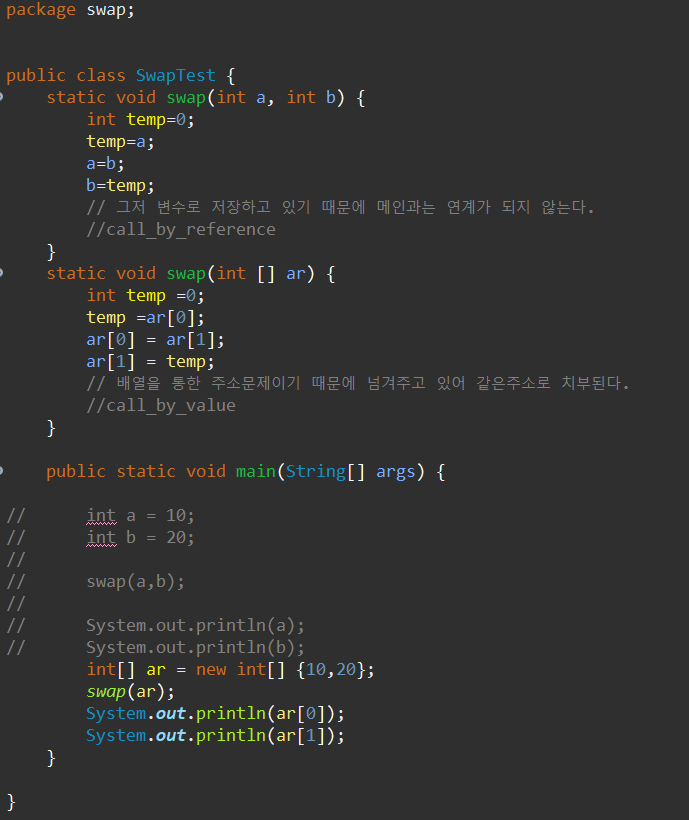
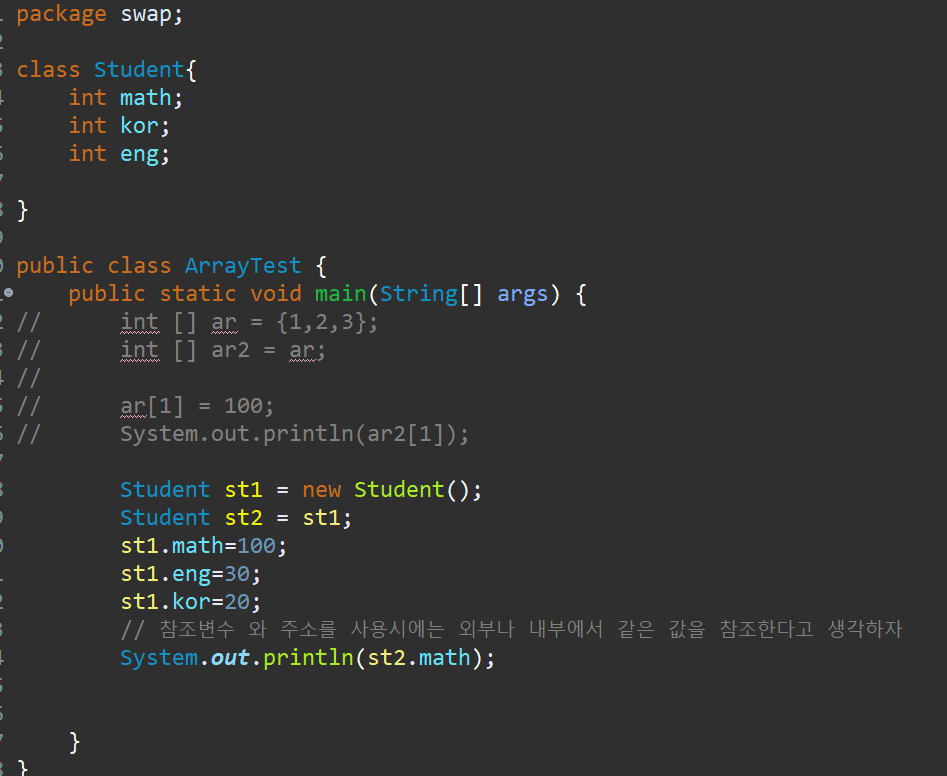
# static(정적인) - 공유되는 공간이라고 생각하자
- 멤버 변수의 종류
1. static이 붙은 변수 : static변수, 클래스 변수, 정적변수
2. static이 안붙은 변수 : 인스턴스 변수
- 멤버 메소드의 종류
1. static이 붙은 메소드 : static메소드, 클래스메소드, 정적메소드
2. static이 안붙은 메소드 : 인스턴스 메소드
- 변수, static메소드는 객체를 생성하지 않아도 사용할 수 있다.
- static 변수는 객체와 상관없이 하나의 저장공간이므로 모든 객체가 공유해서 사용한다.
즉, 모든 객체에서 공통으로 사용되는 것에 static을 붙이는 것을 고려한다.
- static메소드는 인스턴스 변수를 사용할 수 없다.
static메소드가 메모리에서 사용준비가 끝나도 인스턴스 변수는 new를 사용하여 객체를 생성하기
전까지는 사용할 수 없기 때문이다.
- 클래스명.멤버명 으로 사용한다.
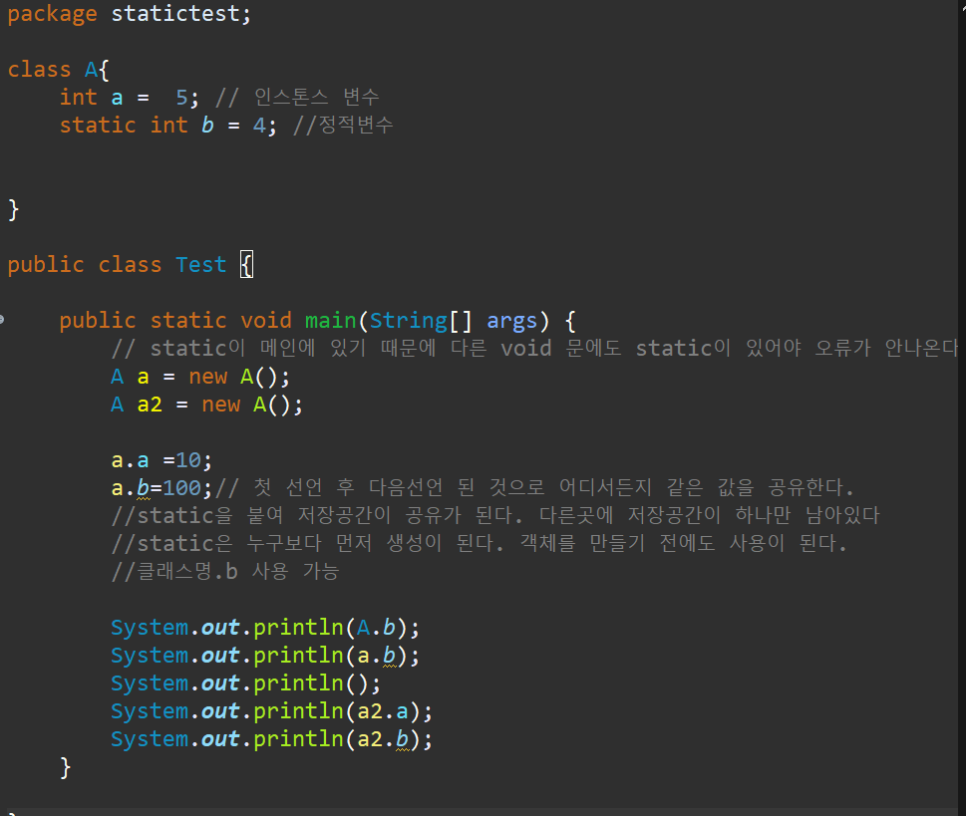
// == 는는 은 주소값을 비교하는 것이다.
//equals 는 해당 값 자체가 같은지 확인하는 것이다.
# Random
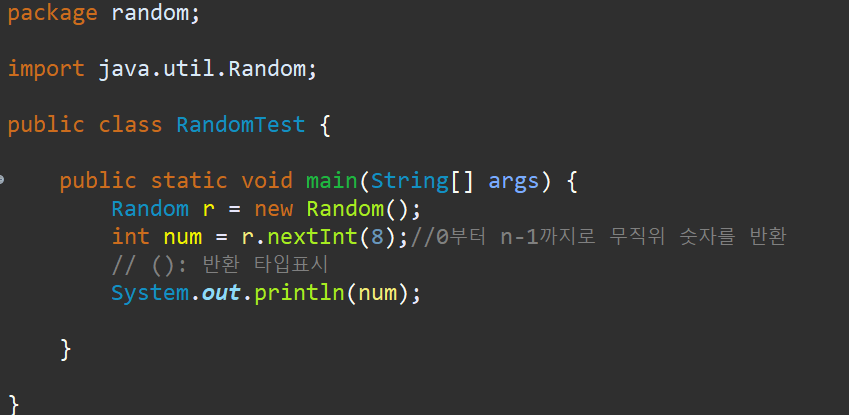
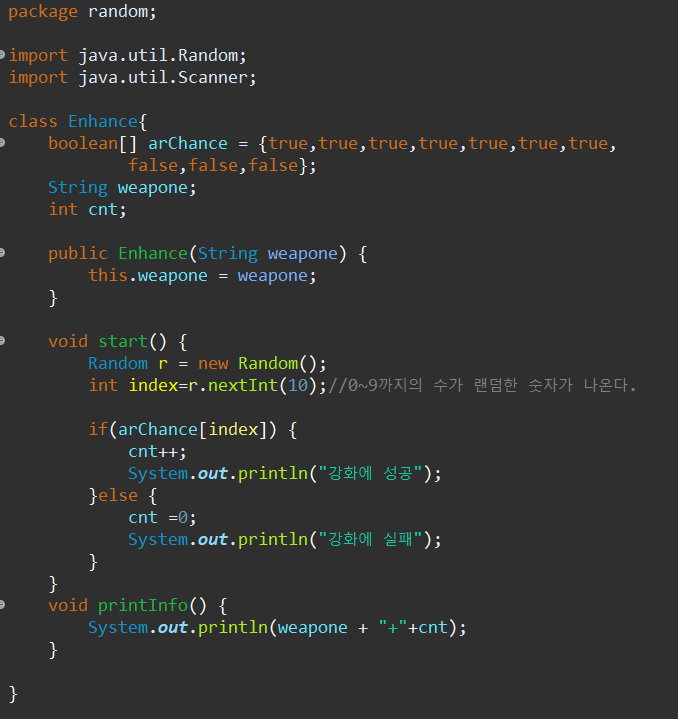
1
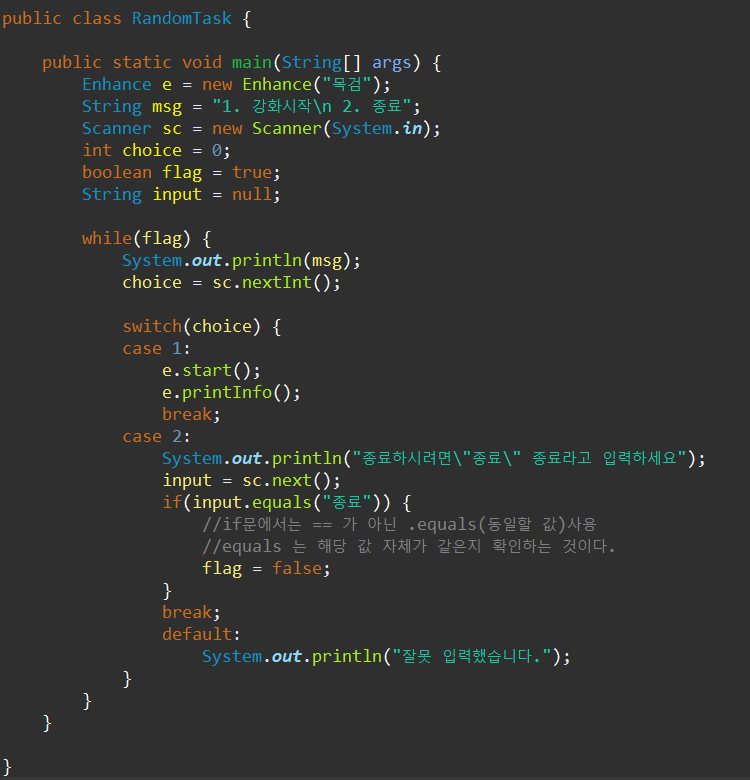
2
# 상속(inheritance)
1. 기존에 선언된 클래스의 맴버(생성자는 제외)를 새롭게 만들 클래스에서 사용하고 싶을 때
상속을 받고 새로운 기능만 추가한다.
2. 여러 클래스 선언 시 멤버가 겹치는 경우, 부모 클래스를 먼저 선언하고 공통맴버를 자식
클래스에게 상속해준다.
- 상속 문법
class Parents {
Parents 멤버
}//부모 클래스
class ChildP extends Parents {
Parents 멤버, Child 멤버
}// 자식 클래스
용하면 된다.
- super : 부모의 참조값, 부모의 멤버에 접근할 때 사용한다.
- super() : 부모 생성자, 자식 클래스 타입의 객체로 필드에 접근할 수 있다.
우리는 객체를 만들 때 자식 생성자만 호출하기 때문에, 자식 필드만 메모리에 할당된다고 생각할 수 있다.\
EX) Chiled ch = new chiled();
그러나 자식 생성자는 항상 부모 생성자를 호출하기 때문에 자식 생성자 호출 시 부모와 생성자도 호출되며
이는 자식의 필드를 초기화 할 때 부모 필드로 초기화 하는 것을 의미한다.
즉, 자식객체를 생성하게 되면 부모 객체도 생성하게 된다.(부모가 먼저 생성) 부모 생성자를 호출하는 방법은 super()를 사용하면 된다.
'Java > Java 학원 복습' 카테고리의 다른 글
자바 접근 권한 제어자, Casting -학원 (0) | 2022.10.03 |
---|---|
자바 상속 - 학원 (0) | 2022.10.03 |
자바 클래스 - 학원 (0) | 2022.10.03 |
자바 학원 필기 7 (0) | 2022.10.03 |
자바 배열 실습문제, 메소드 (1) | 2022.10.03 |