728x90
반응형
✔ 이미지 업로드 API (ServiceImpl)
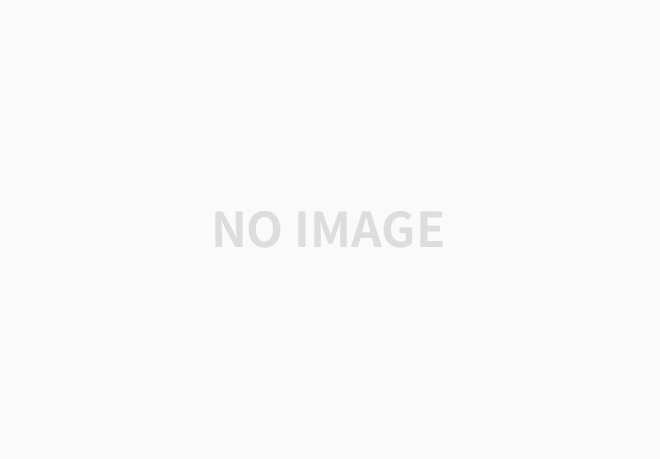
- 기본 틀 (이미지가 없는 경우)
@Override
public JsonElement uploadImageImageVO image) {
StopWatch watch = getStopWatch();
MultiValueMap<String, Object> params = new LinkedMultiValueMap<>();
try {
if (image.getImage() == null) {
return JsonParser.parseString(new Gson().toJson(new ResponseWithMessageVO(ApiCommonCode.ILLEGAL_PARAMETER, "이미지는 필수 항목입니다.")));
}
- 파일 형식 지정해주기 (조건)
// 업로드된 이미지 파일 가져오기
MultipartFile uploadedImage = image.getImage();
// 파일 형식 확인 (jpg와 png만 허용)
String originalFilename = uploadedImage.getOriginalFilename();
if (!originalFilename.toLowerCase().endsWith(".jpg") && !originalFilename.toLowerCase().endsWith(".png")) {
return JsonParser.parseString(new Gson().toJson(new ResponseWithMessageVO(ApiCommonCode.INVALID_IMAGE_FORMAT_EXCEPTION, "이미지 형식이 올바르지 않습니다. JPG와 PNG 형식만 지원됩니다.")));
}
- 파일 크기 지정해주기(조건)
// 파일 크기 확인 (최대 500KB)
long fileSize = uploadedImage.getSize();
long maxSizeInBytes = 500 * 1024; // 500KB
if (fileSize > maxSizeInBytes) {
return JsonParser.parseString(new Gson().toJson(new ResponseWithMessageVO(ApiCommonCode.INVALID_IMAGE_LENGHT_EXCEPTION, "이미지 크기가 허용된 제한을 초과합니다. 최대 크기는 500KB입니다.")));
}
- 이미지 크기 확인 (조건) px, 비율
// 이미지 크기 확인 (가로: 108px 이상, 가로:세로 비율 1:1)
BufferedImage bufferedImage = ImageIO.read(uploadedImage.getInputStream());
int width = bufferedImage.getWidth();
int height = bufferedImage.getHeight();
if (width < 108 || width != height) {
return JsonParser.parseString(new Gson().toJson(new ResponseWithMessageVO(ApiCommonCode.INVALID_IMAGE_SIZE_EXCEPTION, "이미지 크기가 올바르지 않습니다. 가로는 108px 이상이어야 하며, 가로:세로 비율은 1:1이어야 합니다.")));
}
params.add("image", convertFileToByteArrayResource(uploadedImage));
} catch (NullPointerException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
- 저장 부분
String url = uploadUrl + "/image/alim/itemHighli";
log.info(REQUEST_LOG_FORMAT, url);
ResponseEntity<String> response = restTemplate.postForEntity(url, getDefaultHttpEntity(params), String.class);
String body = response.getBody();
watch.stop();
log.info(POST_RESPONSE_LOG_FORMAT, url, params, body, watch.getTotalTimeMillis());
return JsonParser.parseString(response.getBody());
}
log.info(REQUEST_LOG_FORMAT, url);: 이 줄은 요청 URL을 나타내는 정보 메시지를 기록합니다.
'REQUEST_LOG_FORMAT'은 URL을 포함하는 로그 형식의 자리 표시자입니다.
log.info(POST_RESPONSE_LOG_FORMAT, url, params, body, watch.getTotalTimeMillis());: 이 행은
요청 URL, 요청 매개변수(params), 응답 본문(body)을 포함하는 정보 메시지를 기록합니다. , 작업에 소요된 총 시간입니다.
✔ 이미지 업로드 컨트롤러 부분
@RestController
@RequestMapping(value = "api/image", produce ={ MediaType.APPLICATION_JSON_VALUE, MediaType.TEXT_PLAIN_VALUE})
public class ImageController{
private ImageService imageservice;
@Autowired
@Qualifier("imageService")
public void setImageService(ImageService imageService){
this.imageService = imageService;
@PostMapping("/itemHigh")
public String uploadImageAlimtalkItemHighlight(ImageVO image, BindingResult bind){
if(bind.hasErrors()){
throw BindingException.make(bind);
}
return imageService.uploadImageAlimtalkItemHighlight(imageVO).toString();
}
}
}
✔ 응답 코드와 공통 응답 객체
// 공통 응답 객체
@Getter
@NoArgsConstructor
@AllArgsConstructor
public class ResponseVO{
//응답코드
protected String code;
@Override
public String toString() {
return new Gson().toJson(this)
}
}
// 공통 응답 객체
@Getter
public class ResponseWithMessageVO extends ResponseVO{
//응답코드
private String message;
public ResponseWithMessageVO(String code, String message){
super(code);
this.message = message;
}
@Override
public String toString(){
return new Gson().toJson(this);
}
반응형
'업무 기록 > API' 카테고리의 다른 글
API 원격 서버 이미지 업로드(IP동시, Jsch, sftp) (0) | 2023.07.12 |
---|---|
Spring API 이미지 자동 삭제 (0) | 2023.06.18 |
Spring 이미지 업로드 (파일생성, 난수, 경로 설정) (0) | 2023.06.17 |
SpringBoot 메세지 발송/조회 API 설계 2 (Entity, DB, Controller, Service, POSTMAN) (0) | 2023.05.19 |
SpringBoot 메세지 발송/조회 API 설계 1 (요구사항, 사용기술, application.properties, Log4j) (0) | 2023.05.18 |